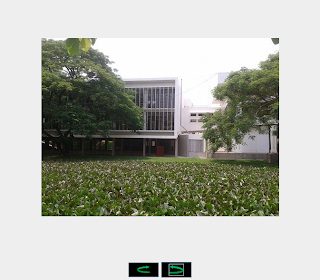
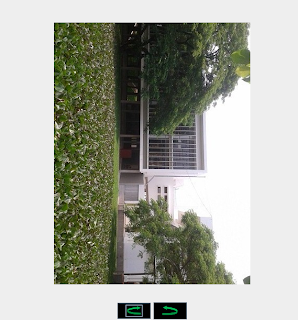
ImageRotate source code:
import
java.awt.*;
import
java.awt.event.*;
import
javax.swing.*;
import
javax.swing.event.*;
import
java.awt.image.*;
class
Rotation extends Canvas implements ImageObserver{
Image
img;
double
radian=0.0;
Dimension ds;
Rotation(){
1 img=getToolkit().getImage("image03.jpg");
2 ds=getToolkit().getScreenSize();
}
3 public void paint(Graphics g){
rotateImage(g);
}
public void rotateImage(Graphics g){
4 Graphics2D
g2d=(Graphics2D)g;
5 int iw=img.getWidth(this);
6 int
ih=img.getHeight(this);
7 int
mX=(int)ds.getWidth()/2;
8 int
mY=(int)ds.getHeight()/2;
9 g2d.translate(mX,mY);
10 g2d.rotate(radian);
11 g2d.drawImage(img,-img.getWidth(this)/2,-img.getHeight(this)/2,iw,ih,this);
}
12 public void rotateClockwise(){
radian+=Math.PI/2;
}
13 public void rotateCounterClockwise(){
radian-=Math.PI/2;
}
}
14 class Main extends JFrame implements
ActionListener{
JButton btrotateClockwise;
JButton btrotateCounterClockwise;
JPanel panel;
Rotation r;
ImageIcon clockwiseIcon;
ImageIcon counterclockwiseIcon;
Main(){
r=new Rotation();
setTitle("Image
Rotate Program");
clockwiseIcon=new
ImageIcon("dir1.png");
btrotateClockwise=new
JButton("",clockwiseIcon);
btrotateClockwise.setBackground(Color.BLACK);
btrotateClockwise.addActionListener(this);
counterclockwiseIcon=new
ImageIcon("dir2.png");
btrotateCounterClockwise=new
JButton("",counterclockwiseIcon);
btrotateCounterClockwise.setBackground(Color.BLACK);
btrotateCounterClockwise.setOpaque(true);
btrotateCounterClockwise.addActionListener(this);
panel=new JPanel();
panel.setLayout(new
FlowLayout());
panel.add(btrotateClockwise);
panel.add(btrotateCounterClockwise);
add(r,BorderLayout.CENTER
);
add(panel,
BorderLayout.SOUTH);
setDefaultCloseOperation(EXIT_ON_CLOSE);
setExtendedState(this.getExtendedState()
| this.MAXIMIZED_BOTH);
setVisible(true);
}
15 public void actionPerformed(ActionEvent
e){
if(e.getSource()==btrotateClockwise)
{
r.rotateClockwise();
r.repaint();
}
else
if(e.getSource()==btrotateCounterClockwise)
{
r.rotateCounterClockwise();
r.repaint();
}
}
}
public class
ImageRotate{
public static void main(String
args[]){
new
Main();
}
}
Code Explanation:
1 Use the getImage(String filename) method to create an image object from the image03.jpg file. You may change the file name to your own image file. The image file has to be placed in the current project folder (where you ImageRotate.java exists).
2 Get the dimension (width and height) of the computer screen so you are able to place the image around the center of the screen.
3 The paint method of the Canvas class is where the drawing begins. It invokes the rotateImage method to draw the image at the specified location when the program opens.
4 Convert the Graphics to Graphics2D. The Graphics2D allows you to move coordinate, rotate, or scale image object.
5 Get the width of the image. The this keyword is used to refer to the ImageObserver. The ImageObserver interface controls the image loading.
6 Get the height of the image.
7 Get the width of the computer screen. The width is divided by 2 to define the x-axis (mX) at the center of the screen.
8 Get the height of the computer screen. The width is divided by 2 to define the y-axis (mY) at the center of the screen.
9 The translate(x,y) method is used to move the original coordinate (0,0) to the coordinate (x,y) at the center of the screen.
10 Rotate the image based on the specified radian value. When the image first loads, we do not want to rotate it so the radian value is 0.0. The radian value changes when the clockwise or counterclockwise button is clicked.
11 Display the image at the coordinate (0,0) in the new drawing area (after moving).
12 The rotateClockwise method is invoked when the clockwise button (btrotateClockwise) is clicked. Every time this button is clicked the radian value increases by half of Math.PI value (3.14).
13 The rotateCounterClockwise method is invoked when the clockwise button (btrotateCounterClockwise) is clicked. Every time this button is clicked the radian value decreases by the same amount as the clockwise button click.
14 The Main class is implemented to load the main interface of the image rotate program. In this program, the interface is divided in to two main areas. One (at the center) is for displaying the image so at the center the Rotation object r is placed in. Another one (at the bottom) to display the clockwise and counterclockwise buttons. These buttons are grouped in the Jpanel.
15 The actionPerformed method of the ActionListener interface is rewritten to enable the actions of the clockwise and counterclockwise buttons.
Hey there! I could have sworn I've been to this site before but after reading through some of the post I realized it's new to me. Anyways, I'm definitely happy I found it and I'll be book-marking and checking back frequently! Optimize meta titles
ReplyDeleteThe best games are built with HTML, CSS, JS today are compiled by us at Impossible Game, and they are completely free for you. Let's play and experience to have the best relaxing moment!
ReplyDelete